之前我们学的基本语法中我们并没有实现程序和人的交互,但是Java给我们提供了这样一个工具类,我们可以获取用户的输入。 java.util.Scanner 是 JDK 5 的新特征,我们可以通过Scanner类来获取用户的输入。
基本语法:
Scanner s = new Scanner(System.in);
通过 Scanner 类的 next() 与 nextLine() 方法获取输入的字符串,在读取前我们一般需要使用 asNext() 与 hasNextLine() 判断是否还有输入的数据。
next():
public class Scanner_Next {
public static void main(String[] args) {
//创建一个扫描对象,通过 System.in 用于接收键盘数据
Scanner scanner = new Scanner(System.in);
System.out.println("请输入(使用 next() 方式接收):");
//判断用户有没有输入字符串
if (scanner.hasNext()){
//使用 next() 接收用户的输入,这句一旦执行,程序会一直等待用户输入完毕
String s = scanner.next();
System.out.println(s);
}
//凡是属于 IO 流的类,如果不关闭就会一直占用资源
scanner.close();
}
}
nextLine():
public class Scanner_NextLine {
public static void main(String[] args) {
//创建一个扫描对象,通过 System.in 用于接收键盘数据
Scanner scanner = new Scanner(System.in);
System.out.println("请输入(使用 nextLine() 方式接收):");
//判断用户有没有输入字符串
if (scanner.hasNextLine()){
String s = scanner.nextLine();
System.out.println(s);
}
}
}
public class Scanner_NotIf {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("请输入(没有 if() 判断) 方式接收):");
//判断用户有没有输入字符串
String s = scanner.nextLine();
System.out.println(s);
}
}
public class Scanner_NextInt_NextFloat {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("请输入整数:");
if (scanner.hasNextInt()){
int i = scanner.nextInt();
System.out.println(i);
} else {
System.out.println(scanner.nextLine() + ",不是整数!");
}
System.out.println("请输入小数:");
if (scanner.hasNextFloat()){
float f = scanner.nextFloat();
System.out.println(f);
} else {
System.out.println(scanner.nextLine() + ",不是小数!");
}
scanner.close();
}
}
public class Scanner_Exer {
public static void main(String[] args) {
//我们可以输入多个数字,并求其总和与平均数,没输入一个数字用回车确认,通过输入非数字来结束输入并输出执行结果
Scanner scanner = new Scanner(System.in);
//AA和
double sum = 0;
//AA计算输入了多少个数字
int total = 0;
System.out.println("请输入数字:");
//通过循环判断是否还有输入,并在里面对每一次进行求和统计
while (scanner.hasNextDouble()) {
double d = scanner.nextDouble();
sum += d;
// total = total + 1;
// total = ++total; 不赋值的话 total++ 和 ++total 效果是一样的
total += 1;
System.out.println("和:" + sum);
System.out.println("平均数:" + sum / total);
System.out.println("计算输入了" + total + "个数字");
}
scanner.close();
}
}
语句与语句之间,框与框之间是按从上到下的顺序进行的,它是由若干个依次执行的处理步骤组成的,它是任何一个算法都离不开的一种基本算法结构。
public class SequenceStructure {
public static void main(String[] args) {
System.out.println("顺序结构1");
System.out.println("顺序结构2");
}
}
我们很多时候需要去判断一个东西是否可行,然后我们才去执行,这样一个过程在程序中用 if 语句来表示
语法:
if(布尔表达式){
//如果布尔表达式为 true 将执行的语句
}
public class If {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("请输入:");
String s = scanner.nextLine();
if (s.equals("hello")){
System.out.println(s);
}
System.out.println("end");
scanner.close();
}
}
有个需求,公司要收购一个软件,成功了,支付100万,失败了,自己找人开发。这样的需求用一个 if 就搞不定了,我们需要有两个判断,需要一个双选择结构,所以就有了 if-else 结构。
语法:
if(布尔表达式){
//如果布尔表达式为 true 将执行的语句
} else {
//如果布尔表达式为 false 将执行的语句
}
public class IfElse {
public static void main(String[] args) {
//考试分数大于 60 就是及格,小于 60 就不及格
Scanner scanner = new Scanner(System.in);
System.out.println("请输入考试分数:");
int score = scanner.nextInt();
if (score >= 60){
System.out.println("及格");
} else {
System.out.println("不及格");
}
scanner.close();
}
}
我们发现刚才的代码不符合实际情况,真实的情况可能存在 ABCD ,存在区间多级判断。比如 90~100 就是 A , 80~90 就是 B ……等等,在生活中我们很多时候的选择也不仅仅只有两个,所以我们需要一个多选择结构来处理这类问题。
语法:
if(布尔表达式1){
//如果 布尔表达式1 为 true 将执行的语句
} else if(布尔表达式2){
//如果 布尔表达式2 为 true 将执行的语句
} else if(布尔表达式3){
//如果 布尔表达式 3为 true 将执行的语句
} else {
//如果以上布尔表达式都不为 true 将执行的语句
}
public class IfElseIfElse {
public static void main(String[] args) {
/*
if 语句至多有 1 个 else 语句, else 语句在所有的 else if 语句之后。
if 语句可以有若干个 else if 语句,它们必须在 else 语句之前。
一旦其中一个 else if 语句检测为 true ,其他的 else if 以及 else 语句都将跳过执行。
*/
Scanner scanner = new Scanner(System.in);
System.out.println("请输入分数:");
int score = scanner.nextInt();
if (score > 90 && score <= 100){
System.out.println("A");
} else if (score > 80 && score <= 90){
System.out.println("B");
} else if (score > 70 && score <= 80){
System.out.println("C");
} else if (score >= 60 && score <= 70){
System.out.println("D");
} else if (score >= 0 && score <= 60){
System.out.println("E");
} else {
System.out.println("请输入正确成绩!!!");
}
scanner.close();
}
}
使用嵌套的 if…else 语句是合法的。也就是说可以在另一个 if 或者 else if 语句中使用 if 或者 else if 语句。可以像 if 语句一样嵌套 else if…else 。
语法:
if(布尔表达式1){
//如果布尔表达式1为 true 将执行的语句
if(布尔表达式2){
//如果布尔表达式2为 true 将执行的语句
}
}
思考:我们需要寻找一个数,在1~100之间(二分法)
public class IfElseIf {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int num = 0;
System.out.println("输入s开始游戏,输入p结束游戏");
String start = scanner.next();
boolean flag = true;
while (start.equals("s")) {
int count = 7;
System.out.println("输入1-100之间的数字");
String str = scanner.next();
if (str.equals("p")) {
System.out.println("游戏结束");
break;
} else if (Integer.parseInt(str) >= 1
&& Integer.parseInt(str) <= 100) {
int answer = Integer.parseInt(str);
Random random = new Random();
int result = random.nextInt(100) + 1;
while (count >= 1) {
boolean r = false;
if (result > Integer.parseInt(str)) {
count--;
System.out.println("你输入的数字过小,你还有" + count + "次机会");
} else if (result < Integer.parseInt(str)) {
count--;
System.out.println("你输入的数字过大,你还有" + count + "次机会");
} else {
r = true;
count--;
System.out.println("恭喜你回答正确");
num++;
break;
}
if (!r) {
System.out.println("再挑一个数字吧");
str = scanner.next();
}
}
} else {
System.out.println("请输入正确的数字");
}
System.out.println("你已经成功答对" + num + "次");
}
}
}
多选择结构还有一个实现方式就是 switch case 语句。
switch case 语句判断一个变量与一系列值中某个值是否相等,每个值称为一个分支。
语法:
switch(expression){
case value :
//语句
break; //可选
case value :
//语句
break; //可选
//你可以有任意数量的 case 语句
default : //可选
//语句
}
switch 语句中的变量类型可以是:
byte、short、int、char
public class SwitchCase_Char {
public static void main(String[] args) {
//switch 匹配一个具体的值,匹配成功就返回当前 case 的值,然后根据是否有 break 语句判断是否继续输出,
//case:穿透如果没有加 break 语句就把下面的语句都输出了
char grade = 'C'; switch (grade) {
case 'A':
System.out.println("优秀");
break;
case 'B':
System.out.println("良好");
break;
case 'C':
System.out.println("中等");
break;
case 'D':
System.out.println("及格");
break;
case 'E':
System.out.println("不及格");
break;
default:
System.out.println("未知等级");
}
}
}
从 JDK7 开始
switch 支持字符串 String 类型
同时 case 标签必须为字符串常量或字面量
public class SwitchCase_String {
public static void main(String[] args) {
String name = "张三";
//JDK7 的新特性,表达式结果可以是字符串
//字符的本质还是数字 //反编译:Java 《== class(字节码文件)
switch (name) {
case "张三":
System.out.println("张三");
break;
case "李四":
System.out.println("李四");
break;
case "王五":
System.out.println("王五");
break;
case "赵六":
System.out.println("赵六");
break;
default:
System.out.println("未知人员");
}
}
}
if 判断区间比较方便,而 switch 在匹配一个具体的值方面更好
反编译:
IDEA 中 Java 项目编译之后输出的路径(class字节码文件所在位置):
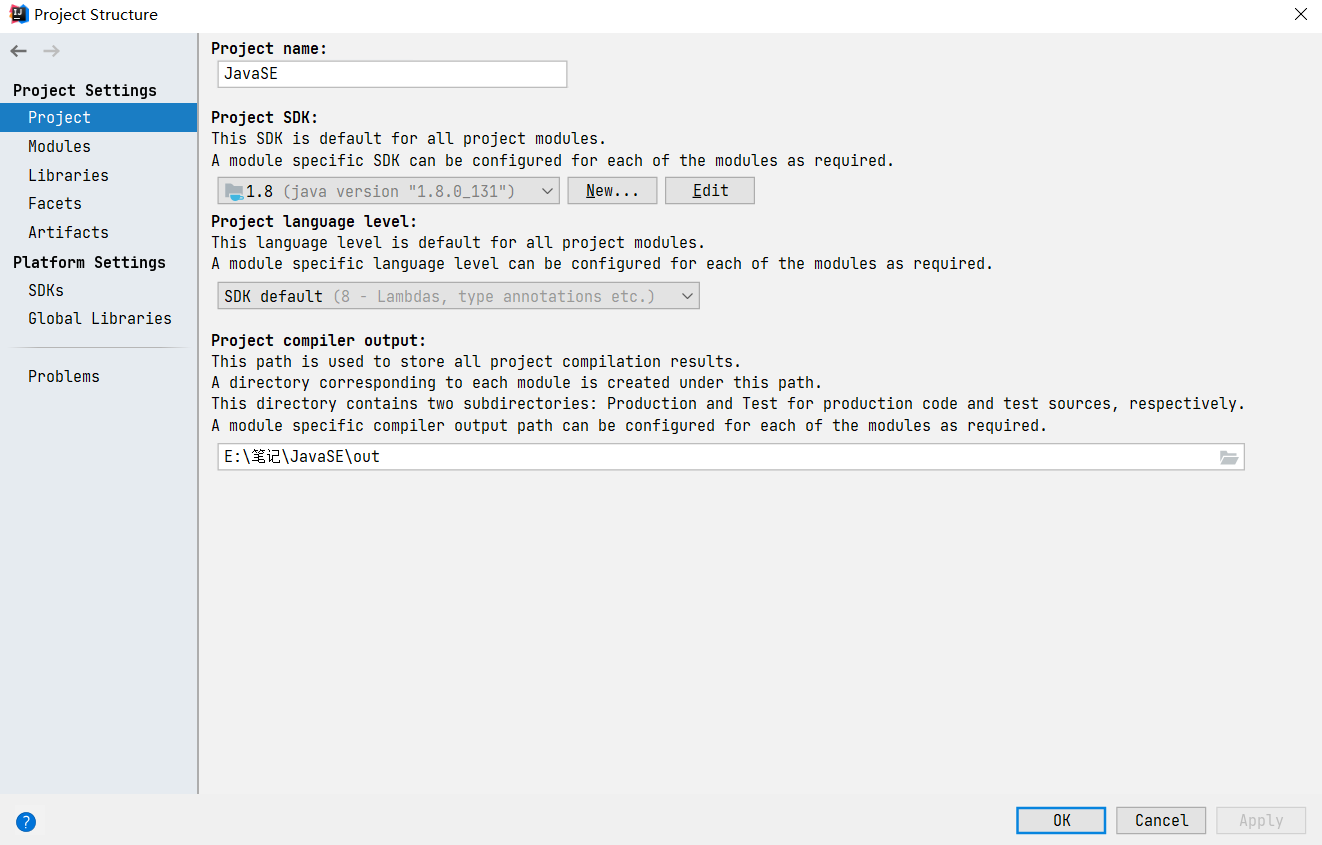
打开文件夹,将 class 文件与 Java 文件放在一起就可以看到反编译之后的文件
//
// Source code recreated from a .class file by IntelliJ IDEA
// (powered by Fernflower decompiler)
//
package com.minami.e_switch_case;
public class SwitchCase_String {
public B_SwitchCase_String() {
}public static void main(String[] args) {
String name = "张三";
byte var3 = -1;
switch(name.hashCode()) {
case 774889:
if (name.equals("张三")) {
var3 = 0;
}
break;
case 842061:
if (name.equals("李四")) {
var3 = 1;
}
break;
case 937065:
if (name.equals("王五")) {
var3 = 2;
}
break;
case 1143448:
if (name.equals("赵六")) {
var3 = 3;
}
}switch(var3) {
case 0:
System.out.println("张三");
break;
case 1:
System.out.println("李四");
break;
case 2:
System.out.println("王五");
break;
case 3:
System.out.println("赵六");
break;
default:
System.out.println("未知人员");
}
}
}
看源码
while是最基本的循环,它的结构为:
while( 布尔表达式 ){
//循环内容
}
只要布尔表达式为true,循环就会一直执行下去。
我们大多数情况是会让循环停止下来的,我们需要一个让表达式失效的方式来结束循环。
public class While {
public static void main(String[] args) {
//输出1~100
int i = 1;
while (i<=100){
System.out.println(i++);
}
}
}
少部分情况需要循环一直执行,比如服务器的请求响应监听等。
循环条件一直为true就会造成无限循环(死循环),我们正常的业务编程中应该尽量避免死循环。会影响程序性能或者造成程序卡死崩溃!
public class While_EndlessLoop {
public static void main(String[] args) {
//死循环--正常的业务编程中应该尽量避免
while (true){
//等待客户端连接--QQ
//定时检查任务--闹钟
}
}
}
思考:计算1+2+3+……+100=?
public class While_Exer {
public static void main(String[] args) {
//计算1+2+3+……+100=?
int i = 1;
int sum = 0;
while (i <= 100) {
sum = sum + i;
i++;
}
System.out.println(sum);
}
}
对于 while 语句而言,如果不满足条件,则不能进入循环。但有时候我们需要即使不满足条件,也至少执行一次。
do…while 循环和 while 循环相似,不同的是, do…while 循环至少会执行一次。
语法:
do{
//代码语句
}while(布尔表达式);
public class DoWhile {
public static void main(String[] args) {
//计算1+2+3+……+100=?
int i = 1;
int sum = 0;
do {
sum = sum + i;
i++;
} while (i <= 100);
System.out.println(sum);
}
}
while 和 do…while 的区别:
while 先判断后执行。 do…while 是先执行后判断!
do…while 总是保证循环体会被至少执行一次!这是它们的主要差别。
public class DoWhile_WhileAndDoWhile {
public static void main(String[] args) {
int i = 0;
while (i < 0){
//不进入循环
System.out.println(i);
i++;
}
System.out.println();
do {
//至少在循环中走一次
System.out.println(i);
i++;
} while (i < 0);
}
}
虽然所有循环结构都可以用 while 或者 do…while 表示,但Java提供了另一种语句 —— for 循环,使一些循环结构变得更加简单。
public class For_WhileAndFor {
public static void main(String[] args) {
//while循环
int i = 1; //初始化条件
while (i <= 100) { //条件判断
//循环体
System.out.println(i);
i+=2; //迭代
}
System.out.println();
//for循环
for (int j = 1; j <= 100; j++) { //初始化条件; 条件判断; 迭代
//循环体
System.out.println(j);
}
/*
关于 for 循环有以下几点说明:
最先执行初始化步骤。可以声明一种类型,但可初始化一个或多个循环控制变量,也可以是空语句。
然后,检查布尔表达式的值。如果为true,循环体被执行。如果为false,循环终止,开始执行循环体后面的语句。
执行一次循环后,更新循环控制变量(迭代因子控制循环变量的增减)。
再次检查布尔表达式,循环执行上面的过程。
*/
//死循环
for (; ; ){ }
}
}
for 循环语句是支持迭代的一种通用结构,是最有效、最灵活的循环结构。
for 循环执行的次数是在执行前就确定的。语法:
for(初始化条件; 布尔表达式; 迭代){
//代码语句
}
练习 1 :计算 0~100 之间的奇数和偶数的和
public class For_Exer1 {
public static void main(String[] args) {
//练习 1 :计算 0~100 之间的奇数和偶数的和
int odd = 0; //奇数
int even = 0; //偶数
for (int i = 0; i <= 100; i++) {
if (i % 2 != 0) {
odd = odd + i;
} else {
even += i;
}
}
System.out.println(odd);
System.out.println(even);
}
}
练习 2 :用 while 或者 for 循环输出 1~100 之间能被 5 整除的数,并且每行输出 3 个
public class For_Exer2 {
public static void main(String[] args) {
//练习 2 :用 while 或者 for 循环输出 1~100 之间能被 5 整除的数,并且每行输出 3 个
for (int i = 1; i <= 1000; i++) {
if (i % 5 == 0) {
System.out.print(i + "\t");
}
if (i % (5 * 3) == 0) {
System.out.println();
}
}
}
}
练习 3 :打印九九乘法表
public class For_Exer3 {
public static void main(String[] args) {
//练习 3 :打印九九乘法表
/*
1*1=1
1*2=2 2*2=4
1*3=3 2*3=6 3*3=9
1*4=4 2*4=8 3*4=12 4*4=16
1*5=5 2*5=10 3*5=15 4*5=20 5*5=25
1*6=6 2*6=12 3*6=18 4*6=24 5*6=30 6*6=36
1*7=7 2*7=14 3*7=21 4*7=28 5*7=35 6*7=42 7*7=49
1*8=8 2*8=16 3*8=24 4*8=32 5*8=40 6*8=48 7*8=56 8*8=64
1*9=9 2*9=18 3*9=27 4*9=36 5*9=45 6*9=54 7*9=63 8*9=72 9*9=81
*/
//1.先打印第一列
//2.把固定的第一列再用一个循环包起来
//3.去掉重复项,j <= i
//4.调整样式
for (int i = 1; i <= 9; i++) {
for (int j = 1; j <= i; j++) {
System.out.print(j + "*" + i + "=" + (i * j) + "\t");
}
System.out.println();
}
}
}
这里先只是见一面,做个了解,之后数组重点使用
JDK5 引入了一种主要用于数组或集合的增强型 for 循环。
语法:
for(声明语句 : 表达式){
//代码语句
}
声明语句:声明新的局部变量,该变量的类型必须和数组元素的类型匹配。其作用域限定在循环语句块,其值与此时数组元素的值相等。
表达式:表达式是要访问的数组名,或者是返回值为数组的方法。
public class ForEach {
public static void main(String[] args) {
int[] arrs = {10, 20, 30}; //定义数组
for (int i = 0; i < arrs.length; i++) {
System.out.println(arrs[i]);
}
System.out.println();
for (int arr : arrs) {
System.out.println(arr);
}
}
}
break 在任何循环语句的主体部分,均可用 break 控制循环流程。 break 用于强行退出循环,不执行循环中剩余的语句。( break 语句也在 switch 语句中使用,用来终止 switch 语句)
public class Break {
public static void main(String[] args) {
int i = 0;
while (i < 100) {
i++;
System.out.println(i); //输出到30
if (i == 30) {
break;
}
}
System.out.println("跳出循环,之后执行这个");
}
}
continue 语句用在循环语句体中,用于终止某次循环过程,即跳过循环体中尚未执行的语句,接着进行下一次是否执行循环的判定
public class Continue {
public static void main(String[] args) {
int i = 0;
while (i < 100) {
i++;
if (i == 30) {
continue;
}
System.out.println(i); //跳过30
}
}
}
关于 goto 关键字(了解即可)
:
的标识符,例如 label:
public class Goto {
public static void main(String[] args) {
//打印 101~150 之间的所有质数(大于1的自然数中,除了1和它本身以外不再有其他因数的自然数)
int count = 0;
outer:for (int i = 101; i <= 150; i++) {
for (int j = 2; j < (i / 2); j++) {
if (i % j == 0) {
continue outer;
}
}
System.out.print(i + "\t");
}
}
}
打印三角形
public class PrintTriangles {
public static void main(String[] args) {
//打印三角形 4行
/*
* 3个空格 1个星
*** 2个空格 3个星
***** 1个空格 5个星
******* 0个空格 7个星
*/
for (int i = 1; i <= 5; i++) {
for (int j = 5; j >= i; j--) {
System.out.print(" ");
}
for (int j = 1; j <= i; j++) {
System.out.print("*");
}
for (int j = 1; j < i; j++) {
System.out.print("*");
}
System.out.println();
}
}
}
手机扫一扫
移动阅读更方便
你可能感兴趣的文章